Bouncy Bouncy Hacky Sack (RC Devlog 2)
Welcome to the first official Rhythm Cataclysm Development log! Today I'll be discussing my struggles with making a bouncy hacky sack and how I overcame them. If you haven't read the previous devlog about what we are making, please do so this will make more sense.
----Our Goal----
The goal is to make the hacky sack *feel* like it physically exists and not just a 2d ball. To accomplish that we can take a note from animators and use it in our game. To animate a ball you have one of three states for it. One: round, two: Squished, and three Stretched. If you Stretch the hacky sack during it's rising and falling it will feel as though it has weight to the hacky sack. Same with squashing it when you hit the hacky sack. It would be replicating what would happen if a ball hit the ground. All the mass of the ball would squish to the floor till the energy got absorbed by the ground. Yet in this case we are hitting the hacky sack sending it back up into the air repeating the stretching again. The only time the hacky sack would be still would be when it reaches the peak of it's height. Here is a quick sketch of what it might look like:
Another cool thing to add to give it more movement would be to give the hacky sack some spin! Technically a real hacky sack wouldn't spin if you play normally, but we want this to look cool, so who cares about realism. For the spin, when the ball is moving left or right. that will determine when it is spinning and in which direction.
Now that we have our goal let's set out and program it!
----Programming----
_Spinning_
Let's do the simplest thing first. To program the spinning hacky sack we need to decide when it should spin and how much. Let's say it spins clockwise when moving to the right and counterclockwise to the left. Now in this game the player hits the hacky sack in one of three places, left, middle, and right. So to have the hacky sack move from one destination to the next, it would only need to move once or twice.
Now lets assume the hacky sack will always move the the next space in the same amount of time. Basically it will take the same time for the hacky sack in reference image 1 to reach "X" or "C". Assuming this, that means the hacky sack will need to travel faster to reach the "C" spot, twice as fast in fact! (Speed = Distance / Time). So if the ball is moving faster when moving the the other side, so should the spinning.
Now that we know what to program let's do it! I'll try to be light on the code and math for this section, and just explain how programming it would look. Good news, programming this will be quite simple anyways!
First, we need to find out which way the hacky sack is moving and how far (one or two spaces). Lucky we can do both of these at the same time. If we take where the hacky sack was on x plane and subtract it from where it is going on the the x plane, we will get the distance it will be traveling, and we get to know the direction biased off if it is negative or not. Great, now we have both of them, except the distance is in pixels and not spaces. To fix that, we just divide the distance by the distance between each spot! This will return 1 or 2 if it moves either one or two spots, and it will be negative if moving to the right, and positive if moving to the left. Now if we just multiply that by how fast we want it to spin and add that to the hacky sack angle each frame, we would achieve our goal! Okay, so I actually went fully in depth about how that was programmed. That was only because this was quite simple, and I won't be doing this for any other sections. To prove, here is the legit code in the game:
var spinRatio = (previousPosition - nextPosition) / keySpotOffset;
image_angle+=spinRatio*5
_Squash_
The squash is slightly easier then the stretch, because you will see this a lot less then the stretch. First, this animation will only occur when the hacky sack is hit by the player. So, once the player presses a key to hit the hacky sack, it will set a flag to start the squish animation. Now, how would a hacky sack look if it were to be hit by a foot? It would flatten out and then slowly reform as it leapt back into the air. Unfortunately we don't know when the hacky sack is hit till it is, thus animating the hacky sack flattening out wont be possible. So, to make up for this we cut straight to the hacky sack flattened once hit. Then as it leaves the foot, it will reform into a circle.
_Stretch_
The stretch is slightly more complex, but let's do it anyways! Our goal for this is to get the hacky sack to stretch in a direction that the balls velocity is moving toward. Velocity is a vector that we can measure the angle of. So using the angle of the velocity we can angle the stretch of the hacky sack. For example if velocity's angle was down (270 or -90 degrees), the stretch of the hacky sack would look like so.
Where if the angle was something like at a slant (315 or -45 degrees), the hacky sack would be slanted like this.
Another way to think of a relation between the two would be to place the velocity vector in the center of the hacky sack.
Where the velocity vector points, so does the hacky sack. Also while using this imaging we can also explain our next goal! We want the hacky sack to be more stretched out the faster it goes. But with this relation they are already entwined with each other. If the velocity vector gets larger, so will the hacky sacks' stretch.
There we go! That is the goal and what we need to do, although I wish it were that simple...
Let me go over some things about the engine I use to program this game. In the engine, when drawing an object to the screen you have a few different fields of values you can change to distort the image of the object you are drawing. Those are but not limited to, X and Y position, X scale, Y scale, Rotation, and a few others I don't need to mention. Once you use that function to draw the image you can't change it any more after one distortion. So, you would have to make sure you did everything you wanted to on the first time you draw the image. I'll cut to the chase here. When drawing the hacky sack to the screen I needed to make more then one change but do them desperately, which this engine wouldn't allow. So here are the steps I wen't through and how I overcame it.
While explaining what my goal here was, I had you over look something important. The hacky sack is spinning first as a ball before it is stretched. Do you see the dilemma? We only have one time we can rotate the hacky sack, yet we need to do it twice, once for the spinning, and once for the stretch angle. So how do I solve this? Surfaces! A surface is a canvas that you can draw things too. The screen you play on is also a surface that is drawn to! But how does this solve the issue? First you draw the rotated hacky sack to a pre-made surface the same size as the hacky sack. Then you use draw that surface to the screen, but stretched and rotated again to achieve what we wanted. Great right? Wrong! I wish it were that easy... What you don't know it that the origin of all surfaces are x=0 y=0, which is the top left corner. That means if I were to rotate that surface the way we had planned it would be way off what we want.
To keep this short on the math, I basically rotated like above and then used vector math to find the new x and y offset to moved it around till the center of the hacky sack is back where it was. Here is my math if you care as much to read it.
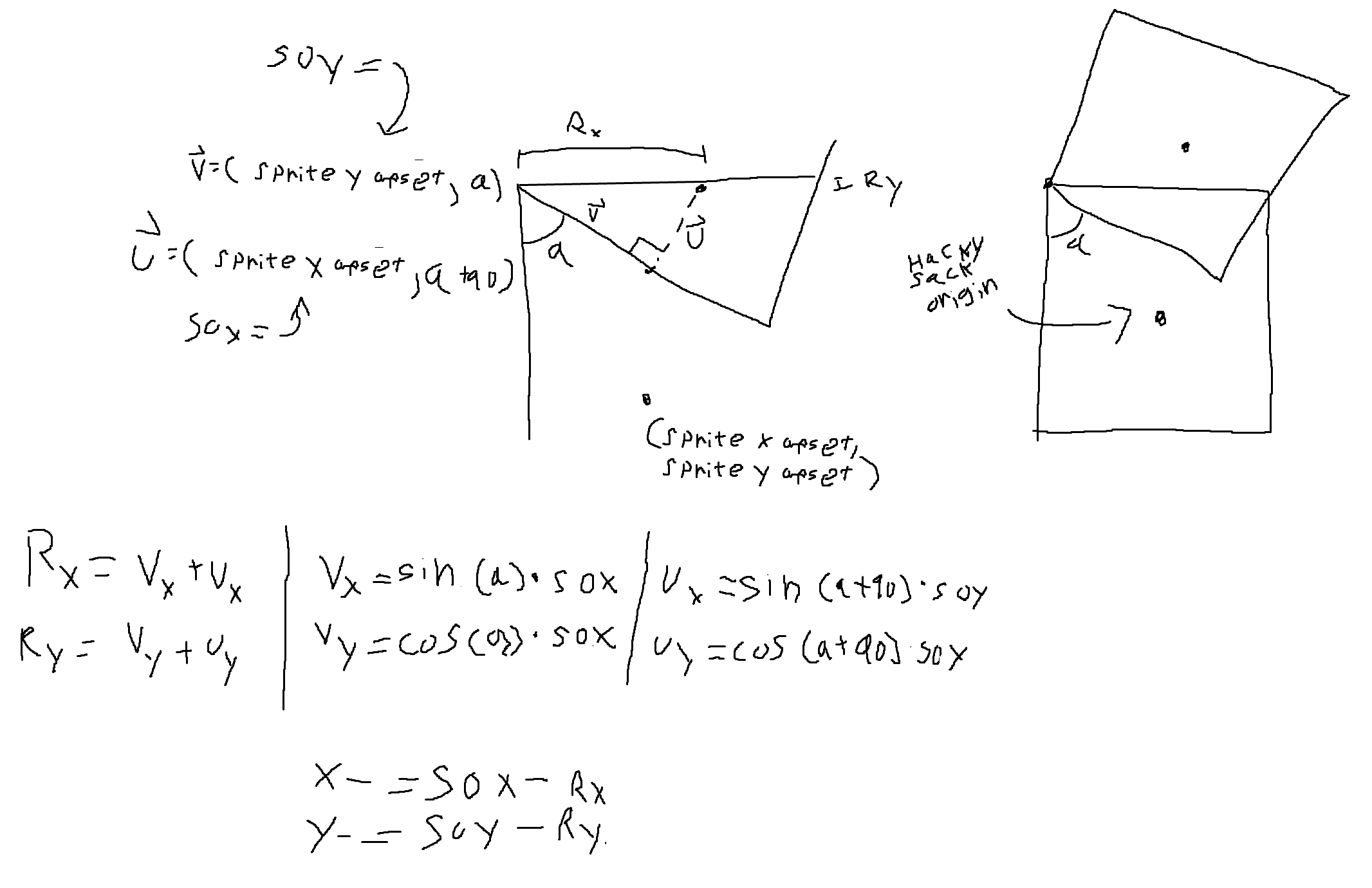
And that's pretty much it for programming the stretching.
------Closing Thoughts------
Wow, you read through the whole thing! I congratulate you, this dev-log was a doozy to write. I'd love to hear your feed back about how I should write these in the future. Would you prefer I go fully in depth about the math and programming, like the first section? Or skim over the programming, but have a good gist of the math still? Or just have me explain the game design and a little insight on how it could be made into a game, but not fully explaining the math behind it.
To be honest any feed back would be great! I would love to hear from you!
And Thank you for reading part 2 of the Rhythm Cataclysm dev-log!
Get Rhythm Cataclysm
Rhythm Cataclysm
a game by Peaceful Illumination submitted for My First Game Jam
More posts
- Getting back into development! & Rhythm Heaven Breakdown (RC Devlog 1)Aug 09, 2020
- Rhythm Cataclysm Dev LogAug 18, 2017
Leave a comment
Log in with itch.io to leave a comment.